When to use gettext() and gettext_lazy() Functions for TranslationAll of these snippets, Django tips and tricks have been tested on real-world Django applications by me. I’m using each of them in my SaaS projects. I wrote this article in a specific manner, less text – more code – more solutions! If you have any issue/error within your Django code – don’t hesitate to share. You will witness the answers to the most frequently asked questions on Django development. Prepare yourself for The collection of useful Django tips from world-class developers!
How to create a custom Django variable that will be accessible anywhere on the website?
(Django variable in base.html)
Navigate to your main Django app(module) folder
and create a new python file, it would be our universal view.py function
# context_processors.py def message_processor(request): if request.user.is_authenticated: no_msgs = request.user.profile.msgs else: no_msgs = 0 return { 'messages' : no_msgs }
Then register your processor under TEMPLATES in your settings.py file:
TEMPLATES = [ { ... 'context_processors': [ ... # custom 'modulename.context_processors.message_processor', ], }, }, ]
And then you will be able to get that variable anywhere in your app as:
{{ message }}
How to get the currently logged in user’s user id in Django?
The current user
is in request
object, you can get it by:
def sample_view(request): current_user = request.user print current_user.id
request.user will give you a User object representing the currently logged-in user. If a user isn’t currently logged in, request.user will be set to an instance of AnonymousUser. You can tell them apart with the field is_authenticated, like so:
if request.user.is_authenticated: # Do something for authenticated users. else: # Do something for anonymous users.
Restrict access to front-end by superuser in Django
Class-based restriction:
create a separate file in the main app’s folder, create a class
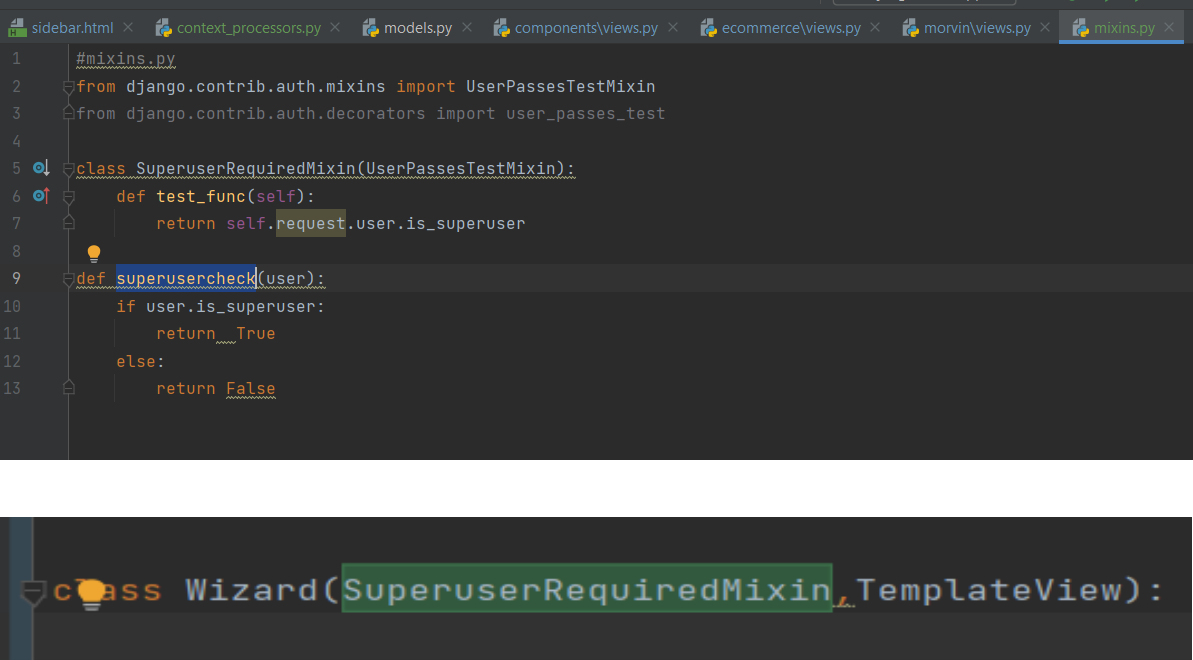
Function-based restrictions:
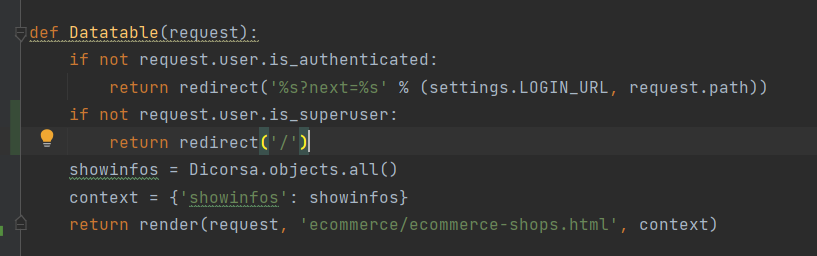
How to get the current Django user on the model. views?
SIMss
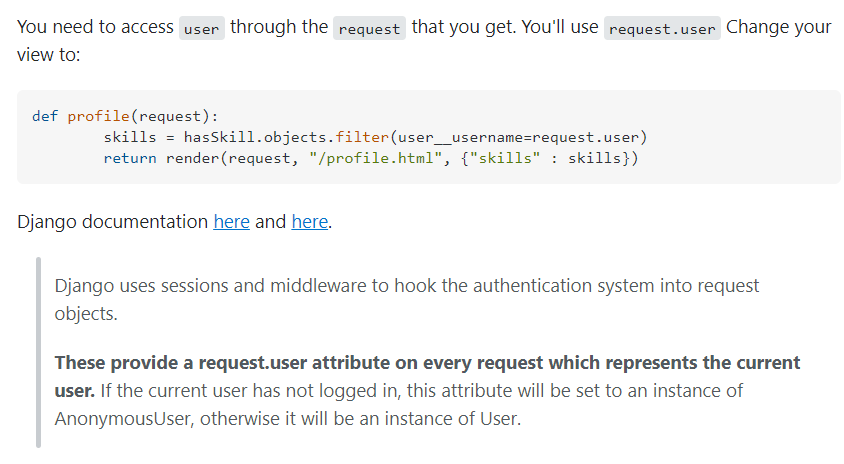
Simply: use the request.user inside the views functions and Dango will return the current user.
How to display DateTime with Django tag on the HTML page and count days since (x days-time ago):
“x Days ago’ template filter in Django?
Simply add the filter tag: timesince, so your code on the HTML page will look like this:
{{ items_updated_at|timesince }}
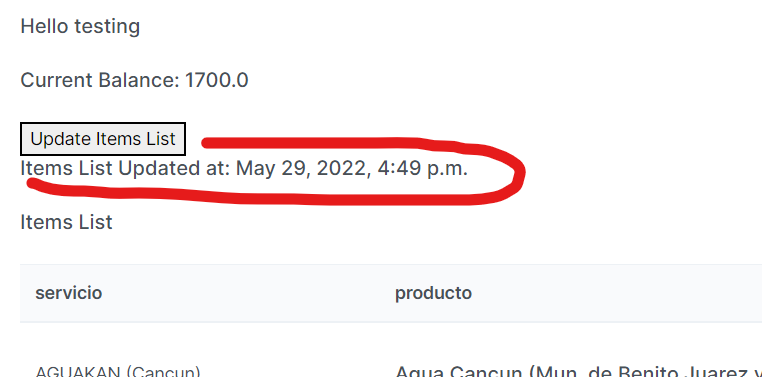
How to retrieve the latest row from the Django Database with Django Models?
Simply add .latest(“row name”) to your Django model query:
Globals.objects.all().latest("items_updated_at")
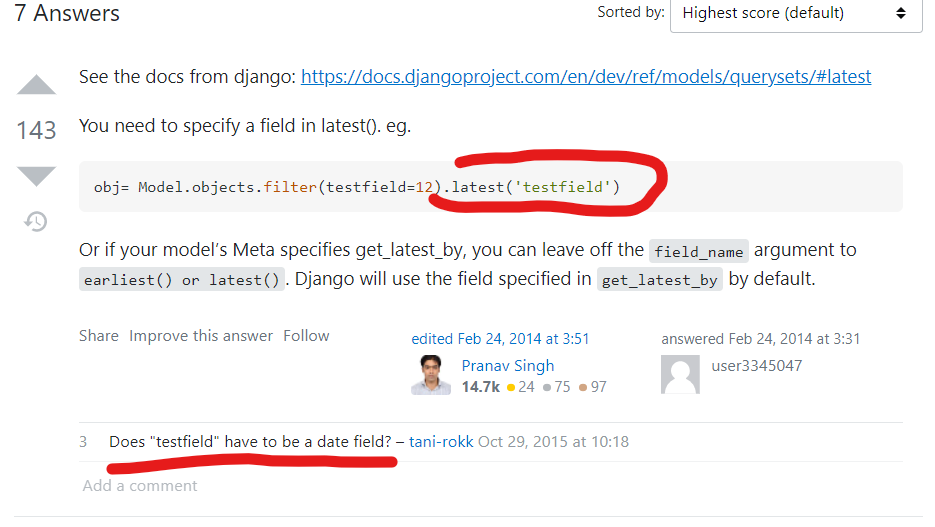
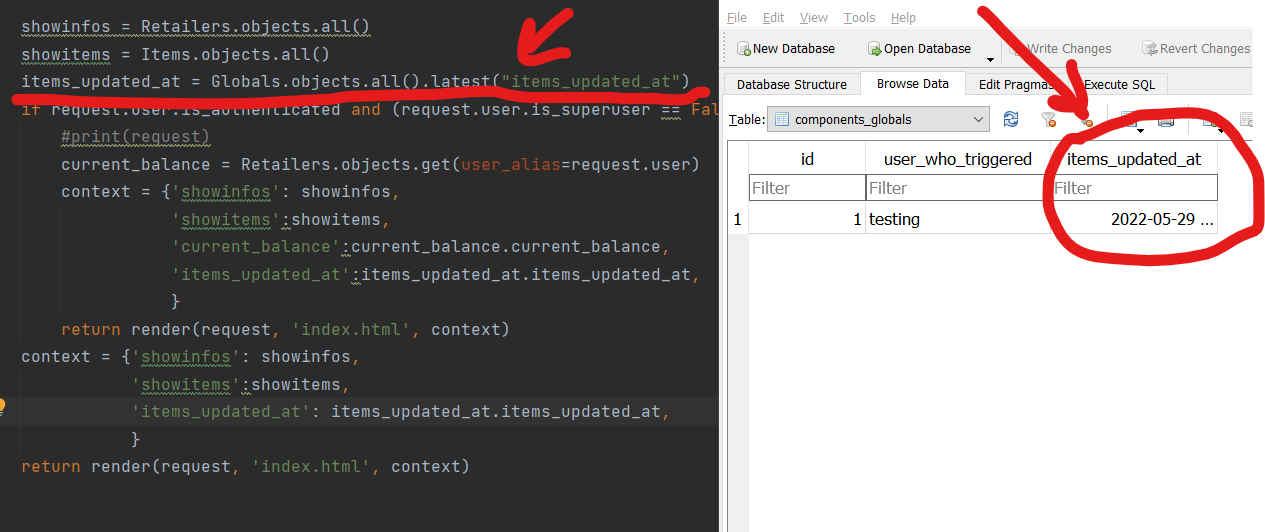
How to populate web form with data from the database by clicking the button on the page
Here we have a {{ previous_transaction_success }} Django Model tag(the information from the database query response), but you can hardcode your data straight forward to the Javascript vars.
On the webpage we have the form with these IDs:
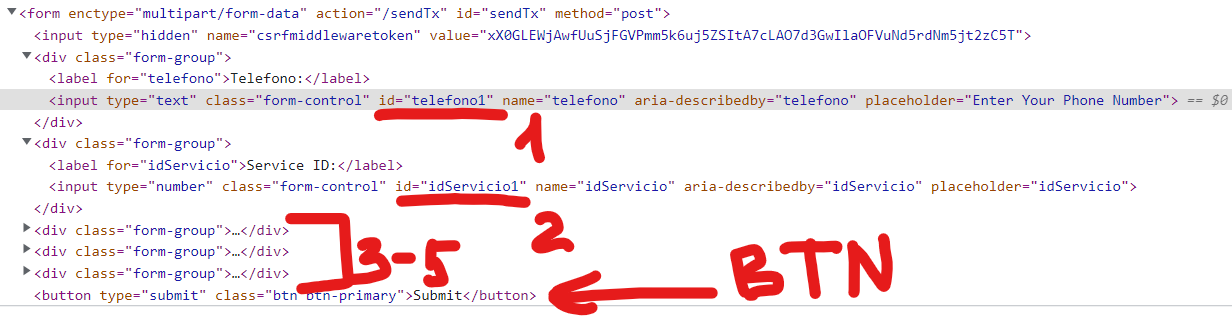
<script> function fill_back_success() { var telefono = {{ previous_transaction_success.telefono }}; var idProducto = {{ previous_transaction_success.idProducto }}; var idServicio = {{ previous_transaction_success.idServicio }}; var montopago = {{ previous_transaction_success.montopago }}; var referencia = {{ previous_transaction_success.referencia }}; document.getElementById("telefono1").value = telefono; document.getElementById("idProducto1").value = idProducto; document.getElementById("idServicio1").value = idServicio; document.getElementById("montoPago1").value = montopago; document.getElementById("referencia1").value = referencia; } </script>
Javascript code will put the values into the web form after we click on the button(the button will trigger the JS function by “onclick=”fill_back_success()””).
Button code:
<button type='submit' onclick="fill_back_success()" class="btn btn-primary">Retry</button>
How to order_by query set in Django, ascending and descending
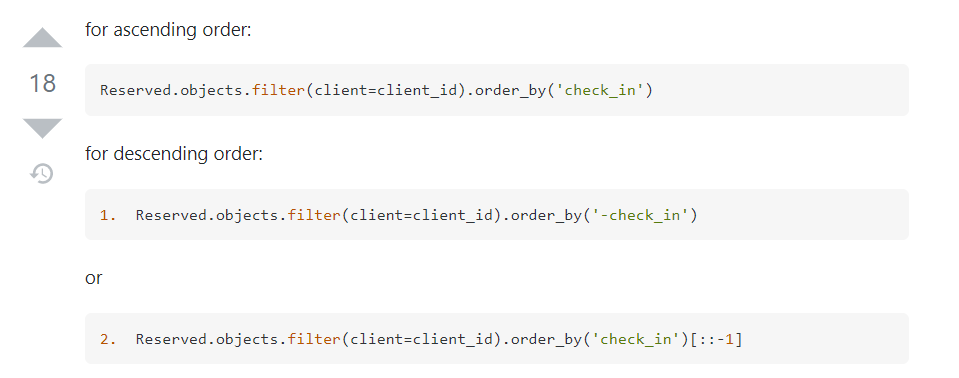
order_by query set in Django for ascending order:
Reserved.objects.filter(client=client_id).order_by('check_in')
order_by query set in Django for descending order:
1. Reserved.objects.filter(client=client_id).order_by('-check_in')
example 2: order_by query set in Django for descending order:
2. Reserved.objects.filter(client=client_id).order_by('check_in')[::-1]
How to use Django multiple template_name in the same View?
Use different template_names on the class-based view:
<code class="hljs language-python">class theTableView(generic.TemplateView): template_name = 'adminTable.html' def get_template_names(self): if self.request.user.is_superuser: template_name = 'goodAdminTable.html' elif self.request.user.is_authenticated: template_name = 'goodUserTable' else: template_name = self.template_name return [template_name]
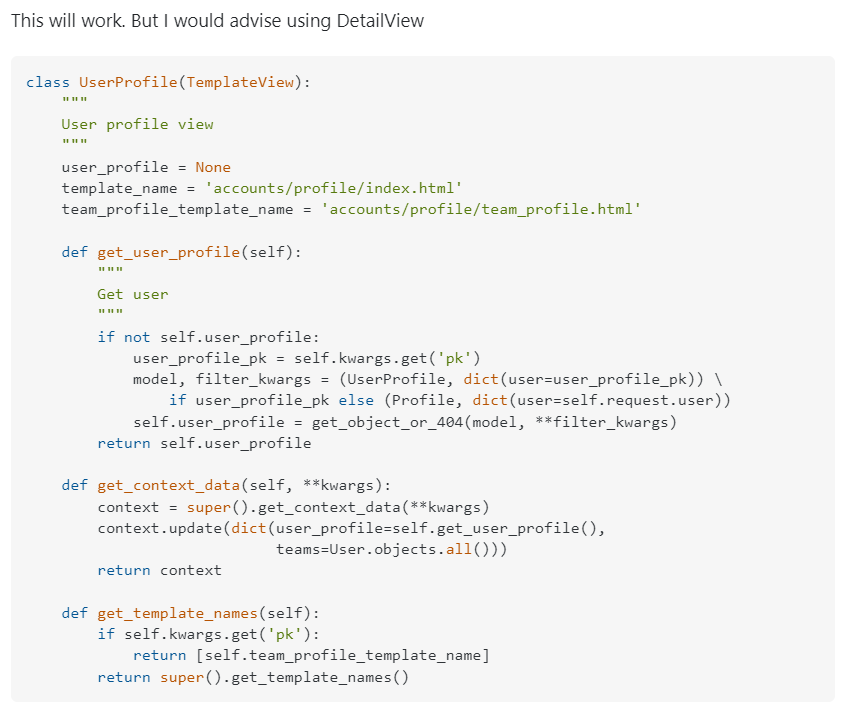
class theTableView(generic.TemplateView): template_name = 'adminTable.html' def get_template_names(self): if self.request.user.is_superuser: template_name = 'goodAdminTable.html' elif self.request.user.is_authenticated: template_name = 'goodUserTable' else: template_name = self.template_name return [template_name]
Use different template_names on the function-based view in Django:
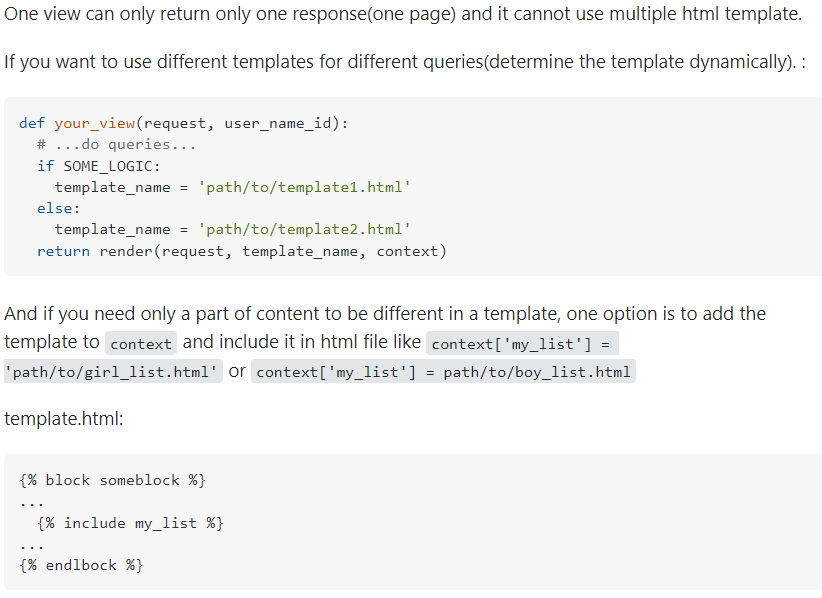
def get_template_names (self): return ["your_template.html",]
How to retrieve the day of the week for the Django database?
To retrieve the day of the week from the Django Database – the data should be saved as Django DateTimeField.
show the day of the week in Django views.py:
use date.weekday() or date.isoweekday()
(will display numbers, the weekday counting from 0, the isoweekday from 1). If you need to display the weekday in English language, just use date.strftime('%A')
This will give you Monday etc.
show the day of the week in Django templates:
pass the variable from your views.py to template.html and use it like this:
{{ date:"l" }} #Monday
How to get a list of column names of the Django model and exclude unnecessary fields from it?
try: #my_model_fields= [field.name for field in Transactions._meta.get_fields()] my_model_fields = [field.name for field in Transactions._meta.get_fields() if field.name not in ('id','bearer_token','bearer_token','json_payload','json_encrypted_payload','response')] # print(my_model_fields) previous_transaction_success = Transactions.objects.filter( user_alias=request.user.username, status='01').order_by('-date').values(*my_model_fields)
How to set the custom default language for Django? (i18n compatible)
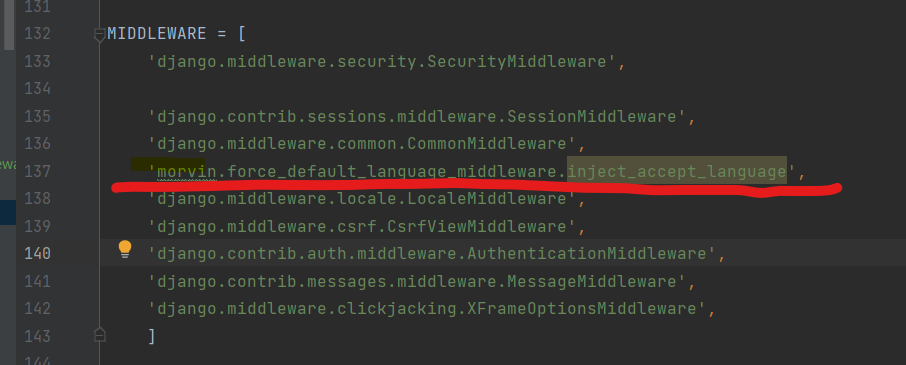
You need to create a custom middleware function/class and apply it to “MIDDLEWARE” in your Django app’s settings.py file. First: create the file with middleware code, in my case I have created the file inside my app’s folder. Don’t forget to import the file inside your settings.py.
from contextlib import suppress from django.conf import settings def inject_accept_language(get_response): """ Ignore Accept-Language HTTP headers. This will force the I18N machinery to always choose - Ukrainian for the main site - ADMIN_LANGUAGE_CODE for the admin site as the default initial language unless another one is set via sessions or cookies. Should be installed *before* any middleware that checks request.META['HTTP_ACCEPT_LANGUAGE'], namely `django.middleware.locale.LocaleMiddleware`. """ admin_lang = getattr(settings, 'ADMIN_LANGUAGE_CODE', settings.LANGUAGE_CODE) def middleware(request): # Force Ukrainian locale for the main site lang = admin_lang if request.path.startswith('/admin') else 'es' accept = request.META.get('HTTP_ACCEPT_LANGUAGE', []).split(',') with suppress(ValueError): # Remove `lang` from the HTTP_ACCEPT_LANGUAGE to avoid duplicates accept.remove(lang) accept = [lang] + accept request.META['HTTP_ACCEPT_LANGUAGE'] = f"""{','.join(accept)}""" return get_response(request) return middleware
This solution is tested and works with Django 3.2, but I am sure it can be tweaked for any version of Django. Please don’t forget that you can write your middleware as a Class as well.
The order of Django model inner classes and standard methods
-
The order of model inner classes and standard methods should be as follows (noting that these are not all required):
- All database fields
- Custom manager attributes
class Meta
def __str__()
def save()
def get_absolute_url()
- Any custom methods
-
If
choices
is defined for a given model field, define each choice as a list of tuples, with an all-uppercase name as a class attribute on the model. Example:class MyModel(models.Model): DIRECTION_UP = 'U' DIRECTION_DOWN = 'D' DIRECTION_CHOICES = [ (DIRECTION_UP, 'Up'), (DIRECTION_DOWN, 'Down'), ]
How to properly translate Django forms, models fields
In models.py import gettext_lazy as _
example field:
country_code = models.CharField(_("Country code"),max_length=5, default="+52")
don’t forget to ignore folders you don’t want to translate
commands:
django-admin makemessages -l es --ignore=venv django-admin compilemessages --ignore=venv
After the first command: navigate to your .po file and manually translate every field’s title/label.
tip: if you want to translate the part of the string, for example, you have: print(f’_(“this text must be translated by the gettext Django”) {somevar}’) – it will not work. It would be best if you folded the string that you want to translate with the variables you want to present, the right code would be:
print(_("this text must be translated by the gettext django")+f"{somevar}")
What’s the difference between gettext and gettext_lazy in Django?
gettext_lazy(): should be used in fields, verbose_name, help_text, methods short_description of models.py, labels, help_text, empty_label of forms.py, verbose_name of apps.py. gettext(): should be used in views.py and other modules similar to view functions that are executed during the request process. credits to (https://nsikakimoh.com/blog/gettext-and-gettext_lazy-functions-in-django#difference-between-gettext-and-gettext_lazy-functions-in-django)
How to fake/ignore the Django migration?
python manage.py migrate app --fake
*the app’s migration will be faked, which means it will not affect the database and will be ignored. This is very useful when you remove something by mistake and your database doesn’t allow you to easily make changes, to fix everything directly(for example – google cloud spanner).
Best practices on how to create the custom model’s Manager()?
If you already have a Django model and you want to create a custom manager for it, don’t forget to add an “OG” objects field to your model, otherwise, you will end up with the error. So the logic is that if you have created a custom manager for your model, it will interfere with the model_name.objects until you declare the default objects
field for it. Example:
# Creating a custom manager for SomeCompanies model! class ListCompanies(models.Manager): def companies(self,custom_company_name): return super().get_queryset().filter(company_name=custom_company_name) class SomeCompanies(models.Model): company_name = models.CharField(_("Company Name"), max_length=50, default="default") # get_companies_by_names= ListCompanies() # you have added the new Manager to your existing model of `SomeCompanies` objects = models.Manager() # this is crucial, because you have add `get_companies_by_name` manager
In your view, you can use your newly created manager:` x = SomeCompanies.get_companies_by_names.companies(‘MyBossComp’) `. This is equal to
SomeCompanies.objects.filter(company_name=’MyBossComp’)`
This post is the part of “TIPS” series, if you’re into WordPress development and blogging – take a look at my WordPress Tips.
Friends, do not forget that the best way to learn something is to practice, the best answers to Django questions are on the official Django documentation website
Leave a Reply